If you are looking to unlock 40+ HRIS and ATS integrations with a single API key, check out Knit API. If not, keep reading
Note: This is our master guide on API Pagination where we solve common developer queries in detail with common examples and code snippets. Feel free to visit the smaller guides linked later in this article on topics such as page size, error handling, pagination stability, caching strategies and more.
In the modern application development and data integration world, APIs (Application Programming Interfaces) serve as the backbone for connecting various systems and enabling seamless data exchange.
However, when working with APIs that return large datasets, efficient data retrieval becomes crucial for optimal performance and a smooth user experience. This is where API pagination comes into play.
In this article, we will discuss the best practices for implementing API pagination, ensuring that developers can handle large datasets effectively and deliver data in a manageable and efficient manner. (We have linked bite sized how-to guides on all API pagination FAQs you can think of in this article. Keep reading!)
But before we jump into the best practices, let’s go over what is API pagination and the standard pagination techniques used in the present day.
What is API Pagination
API pagination refers to a technique used in API design and development to retrieve large data sets in a structured and manageable manner. When an API endpoint returns a large amount of data, pagination allows the data to be divided into smaller, more manageable chunks or pages.
Each page contains a limited number of records or entries. The API consumer or client can then request subsequent pages to retrieve additional data until the entire dataset has been retrieved.
Pagination typically involves the use of parameters, such as offset and limit or cursor-based tokens, to control the size and position of the data subset to be retrieved.
These parameters determine the starting point and the number of records to include on each page.
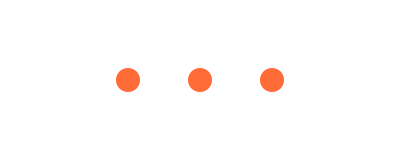
Advantages of API Pagination
By implementing API pagination, developers as well as consumers can have the following advantages -
1. Improved Performance
Retrieving and processing smaller chunks of data reduces the response time and improves the overall efficiency of API calls. It minimizes the load on servers, network bandwidth, and client-side applications.
2. Reduced Resource Usage
Since pagination retrieves data in smaller subsets, it reduces the amount of memory, processing power, and bandwidth required on both the server and the client side. This efficient resource utilization can lead to cost savings and improved scalability.
3. Enhanced User Experience
Paginated APIs provide a better user experience by delivering data in manageable portions. Users can navigate through the data incrementally, accessing specific pages or requesting more data as needed. This approach enables smoother interactions, faster rendering of results, and easier navigation through large datasets.
4. Efficient Data Transfer
With pagination, only the necessary data is transferred over the network, reducing the amount of data transferred and improving network efficiency.
5. Scalability and Flexibility
Pagination allows APIs to handle large datasets without overwhelming system resources. It provides a scalable solution for working with ever-growing data volumes and enables efficient data retrieval across different use cases and devices.
6. Error Handling
With pagination, error handling becomes more manageable. If an error occurs during data retrieval, only the affected page needs to be reloaded or processed, rather than reloading the entire dataset. This helps isolate and address errors more effectively, ensuring smoother error recovery and system stability.
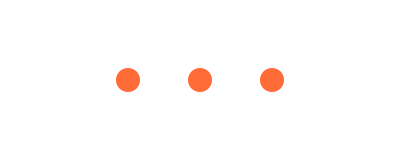
Common examples of paginated APIs
Some of the most common, practical examples of API pagination are:
- Platforms like Twitter, Facebook, and Instagram often employ paginated APIs to retrieve posts, comments, or user profiles.
- Online marketplaces such as Amazon, eBay, and Etsy utilize paginated APIs to retrieve product listings, search results, or user reviews.
- Banking or payment service providers often provide paginated APIs for retrieving transaction history, account statements, or customer data.
- Job search platforms like Indeed or LinkedIn Jobs offer paginated APIs for retrieving job listings based on various criteria such as location, industry, or keywords.
API pagination techniques
There are several common API pagination techniques that developers employ to implement efficient data retrieval. Here are a few useful ones you must know:
- Offset and limit pagination
- Cursor-based pagination
- Page-based pagination
- Time-based pagination
- Keyset pagination
Read: Common API Pagination Techniques to learn more about each technique
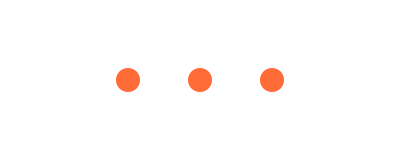
Best practices for API pagination
When implementing API pagination in Python, there are several best practices to follow. For example,
1. Use a common naming convention for pagination parameters
Adopt a consistent naming convention for pagination parameters, such as "offset" and "limit" or "page" and "size." This makes it easier for API consumers to understand and use your pagination system.
2. Always include pagination metadata in API responses
Provide metadata in the API responses to convey additional information about the pagination.
This can include the total number of records, the current page, the number of pages, and links to the next and previous pages. This metadata helps API consumers navigate through the paginated data more effectively.
For example, here’s how the response of a paginated API should look like -
3. Determine an appropriate page size
Select an optimal page size that balances the amount of data returned per page.
A smaller page size reduces the response payload and improves performance, while a larger page size reduces the number of requests required.
Determining an appropriate page size for a paginated API involves considering various factors, such as the nature of the data, performance considerations, and user experience.
Here are some guidelines to help you determine the optimal page size.
Read: How to determine the appropriate page size for a paginated API
4. Implement sorting and filtering options
Provide sorting and filtering parameters to allow API consumers to specify the order and subset of data they require. This enhances flexibility and enables users to retrieve targeted results efficiently. Here's an example of how you can implement sorting and filtering options in a paginated API using Python:
5. Preserve pagination stability
Ensure that the pagination remains stable and consistent between requests. Newly added or deleted records should not affect the order or positioning of existing records during pagination. This ensures that users can navigate through the data without encountering unexpected changes.
Read: 5 ways to preserve API pagination stability
6. Handle edge cases and error conditions
Account for edge cases such as reaching the end of the dataset, handling invalid or out-of-range page requests, and gracefully handling errors.
Provide informative error messages and proper HTTP status codes to guide API consumers in handling pagination-related issues.
Read: 7 ways to handle common errors and invalid requests in API pagination
7. Consider caching strategies
Implement caching mechanisms to store paginated data or metadata that does not frequently change.
Caching can help improve performance by reducing the load on the server and reducing the response time for subsequent requests.
Here are some caching strategies you can consider:
1. Page level caching
Cache the entire paginated response for each page. This means caching the data along with the pagination metadata. This strategy is suitable when the data is relatively static and doesn't change frequently.
2. Result set caching
Cache the result set of a specific query or combination of query parameters. This is useful when the same query parameters are frequently used, and the result set remains relatively stable for a certain period. You can cache the result set and serve it directly for subsequent requests with the same parameters.
3. Time-based caching
Set an expiration time for the cache based on the expected freshness of the data. For example, cache the paginated response for a certain duration, such as 5 minutes or 1 hour. Subsequent requests within the cache duration can be served directly from the cache without hitting the server.
4. Conditional caching
Use conditional caching mechanisms like HTTP ETag or Last-Modified headers. The server can respond with a 304 Not Modified status if the client's cached version is still valid. This reduces bandwidth consumption and improves response time when the data has not changed.
5. Reverse proxy caching
Implement a reverse proxy server like Nginx or Varnish in front of your API server to handle caching.
Reverse proxies can cache the API responses and serve them directly without forwarding the request to the backend API server.
This offloads the caching responsibility from the application server and improves performance.
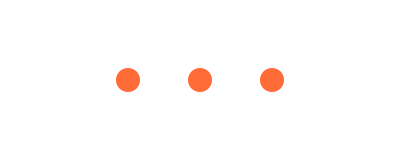
Simplify API pagination
In conclusion, implementing effective API pagination is essential for providing efficient and user-friendly access to large datasets. But it isn’t easy, especially when you are dealing with a large number of API integrations.
Using a unified API solution like Knit ensures that your API pagination requirements is handled without you requiring to do anything anything other than embedding Knit’s UI component on your end.
Once you have integrated with Knit for a specific software category such as HRIS, ATS or CRM, it automatically connects you with all the APIs within that category and ensures that you are ready to sync data with your desired app.
In this process, Knit also fully takes care of API authorization, authentication, pagination, rate limiting and day-to-day maintenance of the integrations so that you can focus on what’s truly important to you i.e. building your core product.
By incorporating these best practices into the design and implementation of paginated APIs, Knit creates highly performant, scalable, and user-friendly interfaces for accessing large datasets. This further helps you to empower your end users to efficiently navigate and retrieve the data they need, ultimately enhancing the overall API experience.
Sign up for free trial today or talk to our sales team